I have this game I am making and I want an input name field panel to pop up on the GameOver screen only when the player achieves a higher score than the previous uploaded score. Of course if there is no personal entry uploaded previously I just want the score to be uploaded. I tried doing it this way to enable the input username panel:
After clearing PlayerPrefs, I tried this code got a Bad Web Request:Should I have used local variables in my code before the Personal Entry was defined or something else?
Should I have used the Leaderboard Search query instead?
No, I just cleared Player Prefs manually. The error then seemed not to appear again after playing the game a few times. Maybe it just was my internet connection? Or Maybe not.
Well then that must have been the case. The leaderboard creator probably did not get properly initialized, 'cause as far as I see from your screenshot, the userGuid parameter was empty.
I am having trouble figuring out how UpdateEntryUserName is used. I don't know much about C# and it would be helpful if you could provide a example scenario and script where you might use UpdateEntryUserName and how it is different from UploadNewEntry. You don't have UpdateEntryUserName in your demo scene for some reason.
<3 Thanks, Let us know if we can contribute to codebase. I moved my project to godot for reasons, I was experimenting with leaderboards, and this project is awesome.
I like how easy this is to use, however I need some help. I am working on a pinball game and getting ready to launch it. I love the idea of using your leader-board. My issue is that when the game is running the player has the option of changing who is playing. When the leader-board is sent an updated score and name the name previously on the leader-board is changed to the new name entered. I assume that there is something set up when the game starts that it only effects updates in that session. Is there a work around?
public void SetLeaderboardEntry(string userName, int score) {
Luckily the FAQ contains an answer to your question:
There may only be one entry per one player. This is done to support features like name editing and deleting an entry in a safe way, such that other people don't mess around with other people's entries. If you do not want this, then disable "Unique Usernames" for your leaderboard and make use of the LeaderboardCreator.ResetPlayer() function during the callback from uploading an entry. Note that after this, a player's previous entry cannot be edited nor deleted by the player themself.
Sorry, I missed that. Works like a charm! One other question, when I decide to upgrade to the Advanced Leaderboard will it keep the same public key and just increase the capacity or will I have to create a new Leaderboard and key?
Hello Dan, the Unique Usernames is a great addition to the leaderboard.
I noticed now that when you have Unique names enabled and I try to call GetLeaderbord with SearchQuery-> Username("Test"), if there is another similar username, lets say ("MyTest" or "Te" or "est") it will return them too.
Would it be possible to make GetLeaderboard(SearchQuery) return the exact UserName (If Unique Username is enabled) instead of all the ones that contain it?
EDIT: This is just a feedback that could be useful for future versions. Not an issue with the leaderboard or hotfix. On my side I could get my intended behaviour with one line of code, but would be nice to get just the unique entry in the future
Hello Dan, Im so happy with this leaderboard youve created. Its so simple to integrate and works perfectly. I just noticed something today:
When using the UpdateEntryUsername() method, the profanity filter does not catch bad words. They are only catched when creating a new entry. Is this intended behaviour?
Currently I have a game that asks the player to input a name for it to be used to update the scores. Im trying to remove this and link the name to the Apple game center nickname. Is there a way to link a GUID to an Apple nickname/gamecenter account so when the user re-installs the game gets the same GUID to update their own entry? (without having to use another DB to save nickname/GUID)
Saving to JSON persistent store doesn't work as Apple removes all files when uninstalling a game. Im also looking for players to connect with the same account from different devices.
Unfortunately, I am not sure if there is a straightforward approach to linking a player's GUID to Apple's services. You would have to handle this through an external server with a database.
Hi Dan! Fantastic tool - thanks so much for making it! =)
Quick question: How would I go about obtaining the rank int of just the newly uploaded entry? I've been racking my brain trying to figure it out and downloaded the demo, but haven't been able to come up with a solution.
This is absolutely amazing! :D Thanks so much but I have one small problem with the extra string choice, for some reason it doesn't showup on my actual leaderboard (in game) in text. Thanks :DDD
Thank you for purchasing an advanced leaderboard! You should receive an email with instructions on how to activate it.
About your second question, just query for more entries. By default, the leaderboard search query is set to get entries from 0 to 100 spots. So increasing "Take" field of the query object should do the job.
Will there be performance problems if I set this value to a large value? For example, 100000! Also, can I further expand the number of people the leaderboard can accommodate?
Hi, I have another question. I use the ByUsername of LeaderboardSearchQuery to query my own ranking. Do you query specific players through userGuid? Otherwise, if there is a player with the same name in the leaderboard, how can I query my own ranking!
Hey I love this its so good and thanks for making it free. Easy to implement too. I use it in my most recent game and will most likely use it in future game jams.
EDIT: I had initially thought that the profanity filter wasn't working as I was in a hurry and implemented it last minute but then I noticed this was not the case. If the profanity filter is on, you type in a profanity and try to update the leaderboard, the console prints a debug log and pauses the preview mode. I thought it had crashed but it only just paused. Just unpause the preview to continue lol. Profanity filter works perfectly thanks Dan
One of the GetLeaderboard function overloads includes a "isInAscendingOrder" bool parameter. Set it to false, and you'll always receive scores highest to lowest scores, no matter the sorting of the leaderboard itself.
I have a problem where when I upload a score, it saves as the first one, then on a different computer with a different name, it overwrites the previous one.
A potential reason for this problem may be that you are requesting 1 entry only by the search query. If not, then I'd like you to message me on Discord (@danqzq) where we can further discuss the problem.
Hi, Amazing tool. I have a question. Let's say a player enters their name and submits a score to the leaderboard. The next time they reach the end of the level how would I populate the "Enter Name" textbox with the name the used previously?
Thanks for checking it out. I would just recommend you save the name locally when they enter it, and load the name into the textbox at the start of the level. I have a video on my YouTube channel that describes different ways of saving data in Unity:
Technically the code you've written here should be working if there is an entry in the leaderboard. I recommend checking out the updated demo scene, which demonstrates how to obtain the ranks of the entries. Its included in the downloads.
Hi thanks for this cool leaderboard tool, I'm having trouble with duplicate names, when I add then im unity it will not update,but in your itch.io page it does respect duplicate names?
There is a an option called "Unique Usernames" for each leaderboard. Enabling it will not allow duplicate names, disabling it will allow duplicate names.
Ok now I have a different problem, when I make a GLBuild the score is set correct and wil move up or down acording to the score, but on my publish build it will only set at index[1] with the same score while the score is different.
Weird thing when I do a local network build it works fine, but when I publish on Unity play , it will sometimes only set at [1],with an old score??
This is not a bug, its a feature. As stated in the FAQ of the page, each player may only have one entry. In your case, the player instance on the local network is different from the one on Unity play. You may be asking why this happens.
I explained why this happens in one of my devlogs in itch.io:
Many people were frustrated about why a single player could have only one entry. Well that is because each player will have a unique identifier attached to them on initialization and it will be stored permanently in PlayerPrefs. With this, players can edit or delete only their own entry. This identifier is also used to determine which entry that is being displayed on the leaderboard belongs to the player. Several people apparently were not satisfied with this, so I made the LeaderboardCreator.ResetPlayer() function. After uploading a new entry, you can call it and the next time the player submits again, instead of overwriting the previous one it should create a new one. Just note that the previous ones would be unable to be changed or deleted afterwards.
Thank you for this tool, it is fantastic. Very much considering the premium version for our project but have a few questions...
- Are there any security measures in place to revent a malicious actor from sending their own data from outside our unity game to the leaderboard (since we only use a public key), could they send a new 'bogus' highscore and get it on the leaderboard? (Or worst case, remove other entries)
- Is it possible for users to 'share' their username across multiple devices (without completely disabling unique usernames)
- Yes there are security measures, however, no leaderboard system is always safe. Even AAA games get hacked entries in their leaderboards. The only way to keep it 100% clean is to regularly monitor it, verify scores and remove potentially hacked entries.
- Technically, yes. There is no direct way of doing it, but you can message me on Discord (username: @danqzq) and we can figure out a way to synchronize user's progress across different devices.
hey Dan, this is really cool! thank you so much >:)
I think I found a bug... When using UploadNewEntry and you upload a duplicate entry while "Unique names" is checked, the entry does not upload. But when you upload the entry with a different name (thats not a duplicate), and then change the name to a duplicate name, the name actually changes...
player 1 creates username: Ben success player 2 creates username: Ben error player 2 creates username: Ben2 success now player 2 changes username to Ben success ...??
What an awesome tool! We used this in our Game Jam game: Neon Shift. Was super easy to use and made our lives much easier. Will definitley check out the advanced leaderboard when improving our game!
When I upload to the leaderboard with ascending order checked it only changes my score if I get a higher score(worse in my game) but not if I get a lower score(better in my game)
I Get the error "[LeaderboardCreator] Uploading entry data failed!" right On the line of uploading new one, not on reset and not on getting the leaderboard, the error is simple on the function UploadNewEntry
When I attempt to add a name that is identical to one that is already on the leaderboard, an error occurs.
Hey Dan, I have a problem. The first time I upload an entry everything works just fine but the second time i can't and get an error saying "Uploading entry data failed!". Everything worked fine 2 days ago and I haven't changed any code. What might be causing this?
Hello! You are calling it correctly, however, before getting the leaderboard you have to wait a certain amount of time, because after the reset - the player's new unique id needs to be fetched from the server to identify as a new player, and all of this does not happen instantly.
I seem to be having the same issue and this doesn't seem to be resolving the issue. I have it written like this; am I not calling the method inside the callback properly?
Hello! You are calling it correctly, however, before getting the leaderboard you have to wait a certain amount of time, because after the reset - the player's new unique id needs to be fetched from the server to identify as a new player, and all of this does not happen instantly.
Thanks for reaching out. I tried using the invoke function to add a delay between the reset and getting the leaderboard however my uploading entry data is still failing.
I updated the Unity package to include a callback argument in the ResetPlayer() function, so call the GetLeaderboard() method inside of it to fully await the reset process.
Hi, Dan! Very nice work and thanks a lot for sharing and keeping it updated! <3
I'm using the tool in an Android game, but for some reason it is only working in the Engine. I build some APKs to test it but it doesn't retrieve the data from my Leaderboard... I followed the video from SamYam and at least the score from the mobile game was reaching the leaderboard... Don't know if I'm missing something.
Yeah I have had people talk to me about the tool not working as intended on Android. I am investigating the problem and I might have found a potential solution, just need to test it and release it.
Hey, I'm dealing with a major bug. It wasn't a problem when I first used this tool. Basically whenever I use upload new entry it replaces a previous entry if its score is higher and if its score is lower it only replaces the name of the previous entry. Don't know what could be causing this, I didn't change the script from when it was working.
Hello, this is not a bug but a feature. Each player by default can only have one entry in the leaderboard. If you do not want this, then call the following function right after submitting a new entry:
is there a way to set it up so it gets the name before it starts the game and after when the game gets a winner it auto puts a score onto the username the player put in before
Yes it is plausible to do so. Just let the user input the name first, then save it via PlayerPrefs or other saving method and load the name value when submitting the score of the player.
This error only occurs on the web app of the tool itself, because each test entry is created with a different unique identifier. This will not happen when you integrate the tool in your game, because each player will have a unique identifier assigned to them when launching your game, and it will remain constant unless PlayerPrefs are cleared. Give it a try by downloading the Unity package with the demo scene.
I just downloaded the leaderboard+demo unity package and after importing the unity package I get these errors that you can see in the screenshot. Can you fix them?
I also tried the leaderboard unity package without the demo. It had the same errors.
I tried the directions you gave exactly but Unity complained that version 3.0 of Newtonsoft Json was not compatible with the version of Unity I was using (2021.3.26f1). I then decided to remove the "@3.0" portion of "com.unity.newtonsoft-json@3.0" that you provided and Unity was able to install the latest version of Newtonsoft Json which is apparently at the time of this post 3.2.1. Just thought I would let you know.
I had the same problem so thanks for the fix!! Before it was overwriting the top spot every time I tried to make a new entry. I had the namespace error about needing newtonsoft. Thank you!! Works great now.
Duplicate names are allowed. Unfortunately if you want unique usernames then you'd need to handle that by yourself by comparing usernames with other entries' usernames. However, allowing only unique names is going to be added as a toggle in the next update.
← Return to Leaderboard Creator
Comments
Log in with itch.io to leave a comment.
I have this game I am making and I want an input name field panel to pop up on the GameOver screen only when the player achieves a higher score than the previous uploaded score. Of course if there is no personal entry uploaded previously I just want the score to be uploaded. I tried doing it this way to enable the input username panel:
And here is the submit score code:
After clearing PlayerPrefs, I tried this code got a Bad Web Request:
Should I have used local variables in my code before the Personal Entry was defined or something else?
Should I have used the Leaderboard Search query instead?
Hello David!
Are you clearing your PlayerPrefs in code before uploading an entry?
No, I just cleared Player Prefs manually. The error then seemed not to appear again after playing the game a few times. Maybe it just was my internet connection? Or Maybe not.
Well then that must have been the case. The leaderboard creator probably did not get properly initialized, 'cause as far as I see from your screenshot, the userGuid parameter was empty.
I am having trouble figuring out how UpdateEntryUserName is used. I don't know much about C# and it would be helpful if you could provide a example scenario and script where you might use UpdateEntryUserName and how it is different from UploadNewEntry. You don't have UpdateEntryUserName in your demo scene for some reason.
Hello there!
As of now, the UploadNewEntry could also be used to alter the username of the player. But since you are curious, here is an example:
Can you help me how to delete all users in the leaderboard, not in the dashboard?
Hey there!
Yes, just message me on Discord.
Wish we could use it with godot.
Godot support is in the works! I am working on support for other development platforms as well, as requested by some people. So stay tuned!
<3 Thanks, Let us know if we can contribute to codebase. I moved my project to godot for reasons, I was experimenting with leaderboards, and this project is awesome.
I like how easy this is to use, however I need some help. I am working on a pinball game and getting ready to launch it. I love the idea of using your leader-board. My issue is that when the game is running the player has the option of changing who is playing. When the leader-board is sent an updated score and name the name previously on the leader-board is changed to the new name entered. I assume that there is something set up when the game starts that it only effects updates in that session. Is there a work around?
public void SetLeaderboardEntry(string userName, int score) {
LeaderboardCreator.UploadNewEntry(leaderBoardKey,userName, score,((msg) =>
{ GetLeaderboard();
}));
}
Hello there!
Luckily the FAQ contains an answer to your question:
There may only be one entry per one player. This is done to support features like name editing and deleting an entry in a safe way, such that other people don't mess around with other people's entries. If you do not want this, then disable "Unique Usernames" for your leaderboard and make use of the LeaderboardCreator.ResetPlayer() function during the callback from uploading an entry. Note that after this, a player's previous entry cannot be edited nor deleted by the player themself.
Sorry, I missed that. Works like a charm! One other question, when I decide to upgrade to the Advanced Leaderboard will it keep the same public key and just increase the capacity or will I have to create a new Leaderboard and key?
Usually I give out a new leaderboard with advanced privileges. But I can upgrade existing leaderboards as well, so it is up to you.
Hello Dan, the Unique Usernames is a great addition to the leaderboard.
I noticed now that when you have Unique names enabled and I try to call GetLeaderbord with SearchQuery-> Username("Test"), if there is another similar username, lets say ("MyTest" or "Te" or "est") it will return them too.
Would it be possible to make GetLeaderboard(SearchQuery) return the exact UserName (If Unique Username is enabled) instead of all the ones that contain it?
EDIT: This is just a feedback that could be useful for future versions. Not an issue with the leaderboard or hotfix. On my side I could get my intended behaviour with one line of code, but would be nice to get just the unique entry in the future
Hello Carlos! I may add this in the future, but I don't think it is a big concern at the moment 😅
Hello Dan, Im so happy with this leaderboard youve created. Its so simple to integrate and works perfectly. I just noticed something today:
When using the UpdateEntryUsername() method, the profanity filter does not catch bad words. They are only catched when creating a new entry. Is this intended behaviour?
Hello there!
This was not intended and I am sorry for that. On a good note, this was just fixed. Thanks for your feedback!
thank you very much! Working now.
On another note, I have a question:
Currently I have a game that asks the player to input a name for it to be used to update the scores. Im trying to remove this and link the name to the Apple game center nickname. Is there a way to link a GUID to an Apple nickname/gamecenter account so when the user re-installs the game gets the same GUID to update their own entry? (without having to use another DB to save nickname/GUID)
Saving to JSON persistent store doesn't work as Apple removes all files when uninstalling a game. Im also looking for players to connect with the same account from different devices.
Unfortunately, I am not sure if there is a straightforward approach to linking a player's GUID to Apple's services. You would have to handle this through an external server with a database.
Dan my man! Thank you Kindly!
Hi Dan! Fantastic tool - thanks so much for making it! =)
Quick question: How would I go about obtaining the rank int of just the newly uploaded entry? I've been racking my brain trying to figure it out and downloaded the demo, but haven't been able to come up with a solution.
Think I finally got it actually! Posting below in case anyone else wants to know! (Or in case I goofed and someone wants to correct me lol)
public void RequestRank(string userName)
{
var query = LeaderboardSearchQuery.ByUsername(userName);
LeaderboardCreator.GetLeaderboard(publicLeaderboardKey, false, query, (rankData) =>
{
rank = rankData.Rank;
});
}
Hello! I used your tool in my game, works like a charm!
Hey real quick I get an error when trying to add using Dan.Main
Hello!
Do you happen to be using Assembly definitions in your code base?
thanks for the reply! I ended up fixing it by just reinstalling the package.
This is absolutely amazing! :D Thanks so much but I have one small problem with the extra string choice, for some reason it doesn't showup on my actual leaderboard (in game) in text. Thanks :DDD
Hello!
Can you share more details on how exactly you're trying to display the extra string?
So sorry to have wasted your time, but I realized my mistake,
Thank You So Much, you're a living legend :)
No need to apologize, its fine. Glad you got it to work :)
Hey I have a question. I want to clear the leaderboard daily How can I automate it in this leaderboard tool??
Hello!
As you may know, you get the leaderboard using this function:
Well, it has another overload:
In place of the "searchQuery", you would put:
That is how you can filter the leaderboard to display daily entries.
Its working. Thanks!
Hi Dan. Please help me! I purchased your premium leaderboard, but I don't know how to use it. It seems to be the same as the free version.
In addition, when I tested the leaderboard, I found that when my rank is after about 100, I will not be able to get my rank alone
My code is:
public static void RequestSelfRank(string key, string userName, Action<bool, int> callback)
{
if (!CheckValid())
{
callback?.Invoke(false, 0);
return;
}
var query = LeaderboardSearchQuery.ByUsername(userName);
LeaderboardCreator. GetLeaderboard(key, false, query, (rankData)=>
{
var success = true;
if (rankData == null || rankData. Length == 0)
{
success = false;
}
var selfRank = 0;
if (success)
{
var self = rankData[0];
selfRank = self.Rank;
}
if (selfRank <= 0)
{
success = false;
}
callback?.Invoke(success, selfRank);
});
}
Hello!
Thank you for purchasing an advanced leaderboard! You should receive an email with instructions on how to activate it.
About your second question, just query for more entries. By default, the leaderboard search query is set to get entries from 0 to 100 spots. So increasing "Take" field of the query object should do the job.
Will there be performance problems if I set this value to a large value? For example, 100000! Also, can I further expand the number of people the leaderboard can accommodate?
1. Well it depends, but you can make a paginated system (e.g display 1000 entries at a time).
2. Not as of yet, but I may make another type of purchasable leaderboard with a higher capacity.
Hi, I just want to get my own ranking, what is the right value for Take! Can you tell me the best practice?
Hi, I have another question. I use the ByUsername of LeaderboardSearchQuery to query my own ranking. Do you query specific players through userGuid? Otherwise, if there is a player with the same name in the leaderboard, how can I query my own ranking!
I would suggest a value of 10000, but display only around 100 entries per load.
Hi Dan, thanks its succed to bring leaderboard on my game, you can check it here guys https://play.google.com/store/apps/details?id=com.isoYo.DeliveryDriver
feel free to comment
Hey I love this its so good and thanks for making it free. Easy to implement too. I use it in my most recent game and will most likely use it in future game jams.
EDIT: I had initially thought that the profanity filter wasn't working as I was in a hurry and implemented it last minute but then I noticed this was not the case. If the profanity filter is on, you type in a profanity and try to update the leaderboard, the console prints a debug log and pauses the preview mode. I thought it had crashed but it only just paused. Just unpause the preview to continue lol. Profanity filter works perfectly thanks Dan
Hello!
Glad you enjoyed it. Can you send me the crash log?
EDIT: Thanks for the clarification!
How do I make it so ascending order goes highest to lowest scor
One of the GetLeaderboard function overloads includes a "isInAscendingOrder" bool parameter. Set it to false, and you'll always receive scores highest to lowest scores, no matter the sorting of the leaderboard itself.
I have a problem where when I upload a score, it saves as the first one, then on a different computer with a different name, it overwrites the previous one.
Hello!
A potential reason for this problem may be that you are requesting 1 entry only by the search query. If not, then I'd like you to message me on Discord (@danqzq) where we can further discuss the problem.
Hi, Amazing tool.
I have a question. Let's say a player enters their name and submits a score to the leaderboard.
The next time they reach the end of the level how would I populate the "Enter Name" textbox with the name the used previously?
Thanks
Hello!
Thanks for checking it out. I would just recommend you save the name locally when they enter it, and load the name into the textbox at the start of the level. I have a video on my YouTube channel that describes different ways of saving data in Unity:
Thank you
Hi Dan, I would like to know the ranking position of a Username.
I tried the following code, but it doesn't find anything.
Can you help me? Thank you very much.
public void LoadRankingPosition()
{
LeaderboardCreator.GetLeaderboard(_leaderboardPublicKey, LeaderboardSearchQuery.ByUsername(_playerUsernameInput.text), RankingPosition);
}
public void RankingPosition(Entry[] entries)
{
_rankingPosition.text = $"{entries[0].RankSuffix()}";
}
Hello!
Technically the code you've written here should be working if there is an entry in the leaderboard. I recommend checking out the updated demo scene, which demonstrates how to obtain the ranks of the entries. Its included in the downloads.
Hello, I am having a problem with my current leaderboard system in WebGL build. I will use this instead of that! Thank you
Hi thanks for this cool leaderboard tool, I'm having trouble with duplicate names, when I add then im unity it will not update,but in your itch.io page it does respect duplicate names?
T.I.A
Kind regards Ronald van Erp
https://play.unity.com/mg/other/glbuildpublish
Hello!
There is a an option called "Unique Usernames" for each leaderboard. Enabling it will not allow duplicate names, disabling it will allow duplicate names.
Ok now I have a different problem, when I make a GLBuild the score is set correct and wil move up or down acording to the score, but on my publish build it will only set at index[1] with the same score while the score is different.
Weird thing when I do a local network build it works fine, but when I publish on Unity play , it will sometimes only set at [1],with an old score??
Hello!
This is not a bug, its a feature. As stated in the FAQ of the page, each player may only have one entry. In your case, the player instance on the local network is different from the one on Unity play. You may be asking why this happens.
I explained why this happens in one of my devlogs in itch.io:
Thank you for this tool, it is fantastic. Very much considering the premium version for our project but have a few questions...
- Are there any security measures in place to revent a malicious actor from sending their own data from outside our unity game to the leaderboard (since we only use a public key), could they send a new 'bogus' highscore and get it on the leaderboard? (Or worst case, remove other entries)
- Is it possible for users to 'share' their username across multiple devices (without completely disabling unique usernames)
Hello!
- Yes there are security measures, however, no leaderboard system is always safe. Even AAA games get hacked entries in their leaderboards. The only way to keep it 100% clean is to regularly monitor it, verify scores and remove potentially hacked entries.
- Technically, yes. There is no direct way of doing it, but you can message me on Discord (username: @danqzq) and we can figure out a way to synchronize user's progress across different devices.
Thank you! Dropped a message
hey Dan,
this is really cool!
thank you so much >:)
I think I found a bug... When using UploadNewEntry and you upload a duplicate entry while "Unique names" is checked, the entry does not upload. But when you upload the entry with a different name (thats not a duplicate), and then change the name to a duplicate name, the name actually changes...
Hello!
I'm having trouble understanding what you are trying to say, but just to clarify, you are using UpdateEntryUsername to change the username, right?
I think I finally understood and it should be fixed in a few minutes once the server update is live.
Cool! thanks
just in case I'll rephrase:
player 1 creates username: Ben
success
player 2 creates username: Ben
error
player 2 creates username: Ben2
success
now player 2 changes username to Ben
success ...??
now there are two "Ben" 's in database
What an awesome tool! We used this in our Game Jam game: Neon Shift. Was super easy to use and made our lives much easier. Will definitley check out the advanced leaderboard when improving our game!
When I upload to the leaderboard with ascending order checked it only changes my score if I get a higher score(worse in my game) but not if I get a lower score(better in my game)
Hello! Working on a fix for this in an upcoming update!
Do you know when the update will release? I'm hoping to add it to a game for the post jam jam
Just released :)
I know this is fixed, but what I would do is just encode the score on the leaderboard as 2147483647 - score
This is how people used to do it before the ascending order sorting feature was added 😂
Great tool! Thx ;)
Absolutely love this tool!
Used it in my game: https://elanmakesgames.itch.io/sandwich-stacker
Thanks so much for making it, and I'll definitely be using it in future projects too :)
I Get the error "[LeaderboardCreator] Uploading entry data failed!"
right On the line of uploading new one, not on reset and not on getting the leaderboard, the error is simple on the function UploadNewEntry
Hello!
I released a new update where this should be fixed if you toggle off the new option named "Unique Usernames".
Hey Dan, I have a problem. The first time I upload an entry everything works just fine but the second time i can't and get an error saying "Uploading entry data failed!". Everything worked fine 2 days ago and I haven't changed any code. What might be causing this?
public void GetLeaderBoard() {
LeaderboardCreator.GetLeaderboard(publicLeaderboardKey, ((msg) => {
int loopLength = (msg.Length < names.Count) ? msg.Length : names.Count;
for (int i = 0; i < loopLength; i++) {
names[i].text = msg[i].Username;
scores[i].text = msg[i].Score.ToString();
}
}));
}
public void SetLeaderBoardEntry(string username, int score) {
LeaderboardCreator.UploadNewEntry(publicLeaderboardKey, username, score, ((msg) => {
GetLeaderBoard();
LeaderboardCreator.ResetPlayer();
}));
}
Here is the code.
Hello! You are calling it correctly, however, before getting the leaderboard you have to wait a certain amount of time, because after the reset - the player's new unique id needs to be fetched from the server to identify as a new player, and all of this does not happen instantly.
Hi Dan!
Nice work on the tool, it really has helped me a lot, but I'm running into a weird issue.
I'm trying to upload a new high score for an existing player, but I get the error "Uploading entry data failed!".
I'm using "LeaderboardCreator.ResetPlayer();" after uploading every score.
Here's my code
Am I missing something?
Hello!
You should call this method inside the callback at last (so it will trigger after the score is submitted).
Hey Dan, big fan of your work.
I seem to be having the same issue and this doesn't seem to be resolving the issue. I have it written like this; am I not calling the method inside the callback properly?
Thanks a lot.
LeaderboardCreator.UploadNewEntry(publicLeaderBoardKey, username, score, ((msg) => {
LeaderboardCreator.ResetPlayer();
GetLeaderboard();
}));
Hello! You are calling it correctly, however, before getting the leaderboard you have to wait a certain amount of time, because after the reset - the player's new unique id needs to be fetched from the server to identify as a new player, and all of this does not happen instantly.
Thanks for reaching out.
I tried using the invoke function to add a delay between the reset and getting the leaderboard however my uploading entry data is still failing.
LeaderboardCreator.UploadNewEntry(publicLeaderBoardKey, username, score, ((msg) => {
LeaderboardCreator.ResetPlayer();
Invoke("GetLeaderboard", 45f);
I updated the Unity package to include a callback argument in the ResetPlayer() function, so call the GetLeaderboard() method inside of it to fully await the reset process.
Hi, Dan! Very nice work and thanks a lot for sharing and keeping it updated! <3
I'm using the tool in an Android game, but for some reason it is only working in the Engine. I build some APKs to test it but it doesn't retrieve the data from my Leaderboard... I followed the video from SamYam and at least the score from the mobile game was reaching the leaderboard... Don't know if I'm missing something.
Does anyone had similar issue?
Hello!
Yeah I have had people talk to me about the tool not working as intended on Android. I am investigating the problem and I might have found a potential solution, just need to test it and release it.
That's awesome!
Thank you very much for your support!
Hi, Dan!
Just reporting that now even the scores from mobile isn't reaching the Leaderboard, don't know if you changed anything server side.
Hey can you reach out to me on Discord? I want you to try out a new package of the tool which should solve this issue. My Discord username is @danqzq.
Hey, I'm dealing with a major bug. It wasn't a problem when I first used this tool. Basically whenever I use upload new entry it replaces a previous entry if its score is higher and if its score is lower it only replaces the name of the previous entry. Don't know what could be causing this, I didn't change the script from when it was working.
Hello, this is not a bug but a feature. Each player by default can only have one entry in the leaderboard. If you do not want this, then call the following function right after submitting a new entry:
LeaderboardCreator.ResetPlayer();
What does it use to detect a different player, cuz these are all from separate instances of the game.
It uses PlayerPrefs
Hey, where do I get my secret key after purchasing the advanced leaderboard
Hello!
I sent you an email with the secret key for activating it, thank you for your purchase! :)
is there a way to set it up so it gets the name before it starts the game and after when the game gets a winner it auto puts a score onto the username the player put in before
That's a good suggestion. will give it a try.
Hello!
Yes it is plausible to do so. Just let the user input the name first, then save it via PlayerPrefs or other saving method and load the name value when submitting the score of the player.
I keep getting an error when I try to upload a new score. "Username already exists" when uploading a second score.
I have a WebGl game and users are using their crypto wallet addresses as usernames which would be unique.
Any suggestions how I can resolve this issue would be cool. Tks.
Hello!
This error only occurs on the web app of the tool itself, because each test entry is created with a different unique identifier. This will not happen when you integrate the tool in your game, because each player will have a unique identifier assigned to them when launching your game, and it will remain constant unless PlayerPrefs are cleared. Give it a try by downloading the Unity package with the demo scene.
I just downloaded the leaderboard+demo unity package and after importing the unity package I get these errors that you can see in the screenshot. Can you fix them?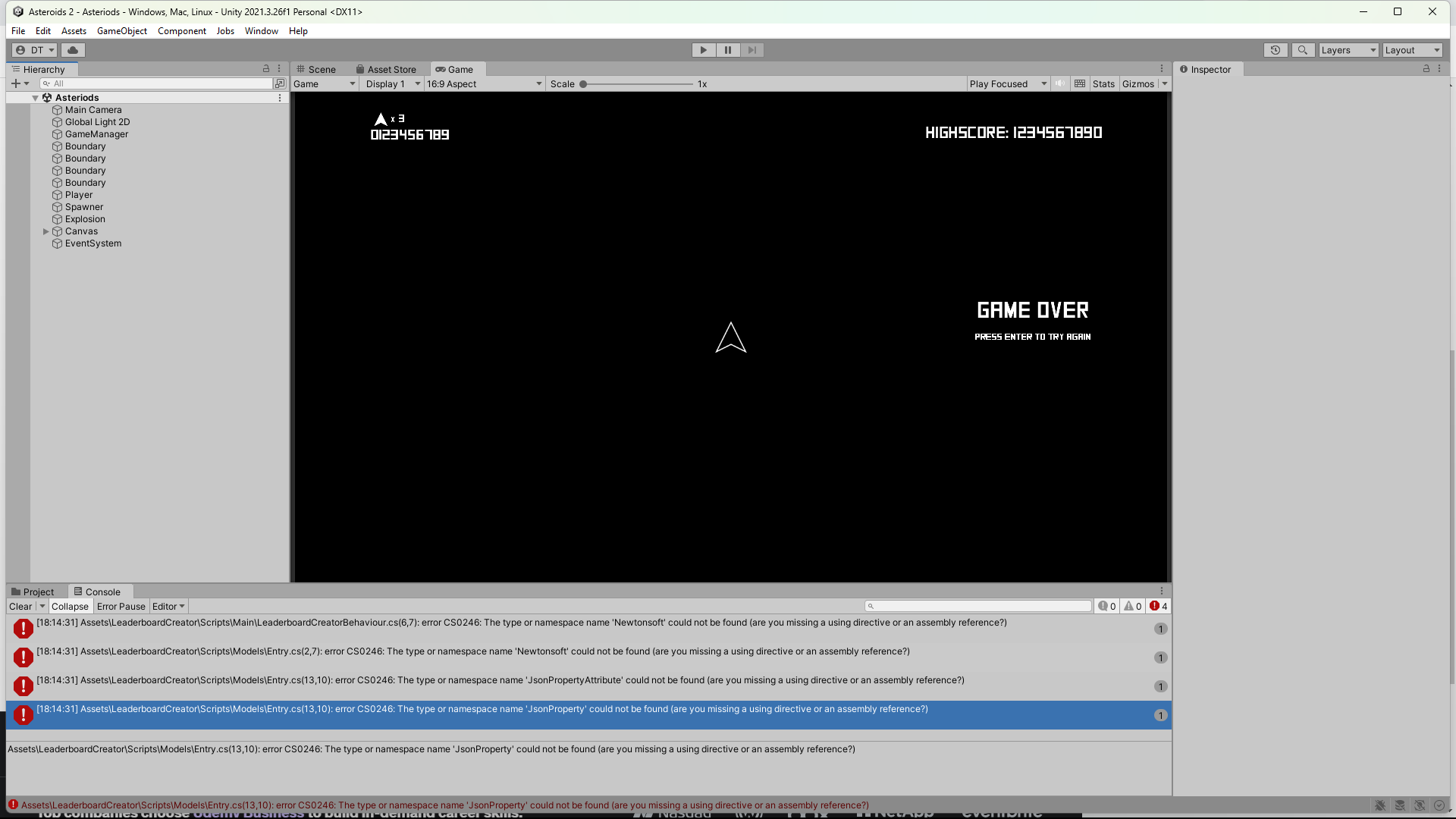
I also tried the leaderboard unity package without the demo. It had the same errors.
Alright, thanks for letting me know!
I had the same problem so thanks for the fix!! Before it was overwriting the top spot every time I tried to make a new entry. I had the namespace error about needing newtonsoft. Thank you!! Works great now.
Hey I purchased the advanced leaderboard, how do I activate it on my leaderboard?
Hello!
Thank you for the purchase! Sent you a secret key for activation!
Hey there. Does this tool handle duplicate names or does the Developer need to write a system to prevent that?
Hello!
Duplicate names are allowed. Unfortunately if you want unique usernames then you'd need to handle that by yourself by comparing usernames with other entries' usernames. However, allowing only unique names is going to be added as a toggle in the next update.